05. Robot Basic Setup
Robot Basic Setup
Let’s build a basic mobile robot model by creating a URDF file and launch it inside an empty Gazebo world.
We can break the effort down into smaller components - a robot base, wheels, and sensors.
For this model, we will create a cuboidal base with two caster wheels. The caster wheels will help stabilize this model. They aren't always required, but they can help with weight distribution, preventing the robot from tilting along the z-axis.
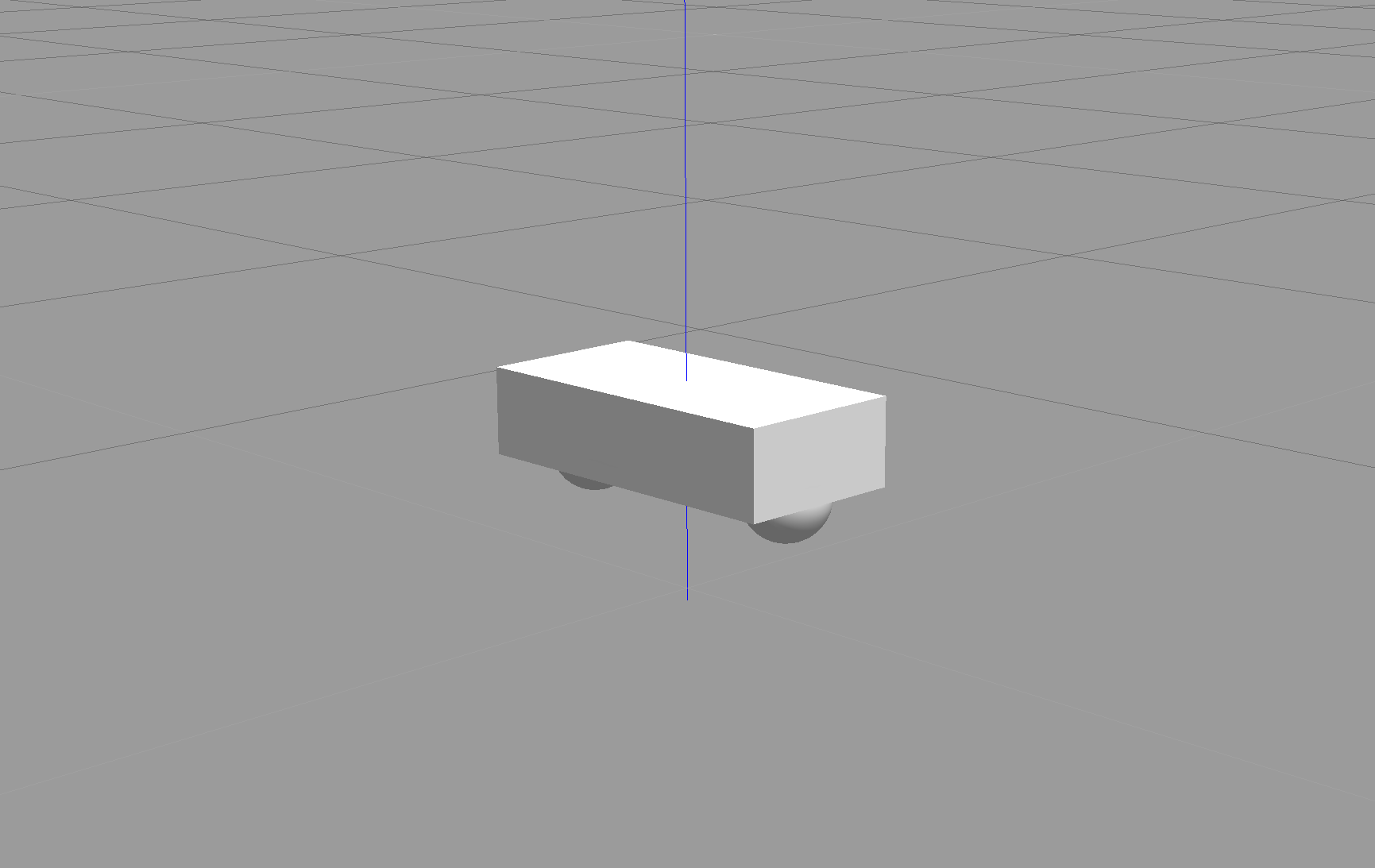
Robot base with two castor wheels
Create the URDF File
1- Create a
urdf
directory in the
my_robot
package
$ cd /home/workspace/catkin_ws/src/my_robot/
$ mkdir urdf
2- Create the robot’s
xacro
file inside the
urdf
directory
$ cd /home/workspace/catkin_ws/src/my_robot/urdf/
$ touch my_robot.xacro
3- Copy the following code into
my_robot.xacro
file
<?xml version='1.0'?>
<robot name="my_robot" xmlns:xacro="http://www.ros.org/wiki/xacro">
<link name="robot_footprint"></link>
<joint name="robot_footprint_joint" type="fixed">
<origin xyz="0 0 0" rpy="0 0 0" />
<parent link="robot_footprint"/>
<child link="chassis" />
</joint>
<link name='chassis'>
<pose>0 0 0.1 0 0 0</pose>
<inertial>
<mass value="15.0"/>
<origin xyz="0.0 0 0" rpy=" 0 0 0"/>
<inertia
ixx="0.1" ixy="0" ixz="0"
iyy="0.1" iyz="0"
izz="0.1"
/>
</inertial>
<collision name='collision'>
<origin xyz="0 0 0" rpy=" 0 0 0"/>
<geometry>
<box size=".4 .2 .1"/>
</geometry>
</collision>
<visual name='chassis_visual'>
<origin xyz="0 0 0" rpy=" 0 0 0"/>
<geometry>
<box size=".4 .2 .1"/>
</geometry>
</visual>
<collision name='back_caster_collision'>
<origin xyz="-0.15 0 -0.05" rpy=" 0 0 0"/>
<geometry>
<sphere radius="0.0499"/>
</geometry>
</collision>
<visual name='back_caster_visual'>
<origin xyz="-0.15 0 -0.05" rpy=" 0 0 0"/>
<geometry>
<sphere radius="0.05"/>
</geometry>
</visual>
<collision name='front_caster_collision'>
<origin xyz="0.15 0 -0.05" rpy=" 0 0 0"/>
<geometry>
<sphere radius="0.0499"/>
</geometry>
</collision>
<visual name='front_caster_visual'>
<origin xyz="0.15 0 -0.05" rpy=" 0 0 0"/>
<geometry>
<sphere radius="0.05"/>
</geometry>
</visual>
</link>
</robot>
We have a single link, with the
name
defined as "chassis", encompassing the base as well as the caster wheels. Every link has specific elements, such as the
inertial
or the
collision
elements. You can quickly review the details of these elements covered in the previous section. The chassis is a cube, whereas the casters are spherical, as denoted by their
<geometry>
tags. Each link (or joint) has an origin (or pose), as well. Every element of that link or joint will have its own origin, which will be relative to the link's frame of reference.
For this base, the casters are included as part of the link for stability. There is no need for any additional links to define the casters, and therefore no joints to connect them. The casters do, however, have
friction
coefficients defined for them. These friction coefficients are set to 0, to allow for free motion while moving.
Launch the Robot
Now that you’ve built the basic robot model, let’s create a launch file to load it inside an empty Gazebo world.
1- Create a new launch file to load the
URDF
model file
$ cd /home/workspace/catkin_ws/src/my_robot/launch/
$ touch robot_description.launch
2- Copy the following code into
robot_description.launch
file
<?xml version="1.0"?>
<launch>
<!-- send urdf to param server -->
<param name="robot_description" command="$(find xacro)/xacro --inorder '$(find my_robot)/urdf/my_robot.xacro'" />
</launch>
To generate the URDF file from the Xacro file, you must first define a parameter,
robot_description
. This parameter will set a single command to use the
xacro package
to generate the URDF from the xacro file.
3- Update the
world.launch
file created earlier so that Gazebo can load the robot
URDF
model
Add the following to the launch file (after
<launch>
):
<!-- Robot pose -->
<arg name="x" default="0"/>
<arg name="y" default="0"/>
<arg name="z" default="0"/>
<arg name="roll" default="0"/>
<arg name="pitch" default="0"/>
<arg name="yaw" default="0"/>
<!-- Launch other relevant files-->
<include file="$(find my_robot)/launch/robot_description.launch"/>
Note: If you have copied your gazebo world from Project 1 then you could skip this step, since you already have
my_robot
in your Gazebo world.
Add the following to the launch file (before
</launch>
):
<!-- Find my robot Description-->
<param name="robot_description" command="$(find xacro)/xacro --inorder '$(find my_robot)/urdf/my_robot.xacro'"/>
<!-- Spawn My Robot -->
<node name="urdf_spawner" pkg="gazebo_ros" type="spawn_model" respawn="false" output="screen"
args="-urdf -param robot_description -model my_robot
-x $(arg x) -y $(arg y) -z $(arg z)
-R $(arg roll) -P $(arg pitch) -Y $(arg yaw)"/>
The
gazebo_ros package
spawns the model from the URDF that
robot_description
helps generate.
Launch
$ cd /home/workspace/catkin_ws/
$ catkin_make
$ source devel/setup.bash
$ roslaunch my_robot world.launch
Note : Launching Gazebo for the first time with a model can take time for everything to load up.
Task Description:
Follow these steps to model a basic robot with a URDF file
Task Feedback:
Great job!